线性布局 (Row、Column)
Row和Column, 即: 水平方向和垂直方向排列子组件;
构造方法
两个轴向:
- mainAxis: 主轴, 即本组件主体的方向
- crossAxis: 纵轴, 即垂直于主轴的方向
Row
主轴: 水平方向
1 | const Row({ |
Column
主轴: 垂直方向
1 | const Column({ |
可以发现构造方法基本一样, 接下来看下具体参数的作用
参数大致含义
- mainAxisAlignment: 表示子组件在主轴(Row为水平方向)的对齐方式, 参考系textDirection
- .start: 表示沿textDirection阅读方向的初始方向对齐
- .end: 和.start正好相反
- .center: 表示居中对齐
- .spaceBetween: 平均分控件之间 0:x:x:0
- .spaceAround: 平均分周围 x:xx:xx:x
- .spaceEvenly: 平均分所有间隙 x:x:x:x
- mainAxisSize: 表示在主轴(Row为水平方向)的占用空间
- .max: 表示尽可能多的占用水平方向的空间,此时无论子 widgets 实际占用多少水平空间,Row的宽度始终等于水平方向的最大宽度;
- .min: 表示尽可能少的占用水平空间,当子组件没有占满水平剩余空间,则Row的实际宽度等于所有子组件占用的的水平空间;
- crossAxisAlignment: 表示子组件在纵轴(Row为垂直方向)的对齐方式, 高度等于子组件高度中最大值, 参考系是verticalDirection
- .start
- .end
- .center
- .stretch
- .baseline
- textDirection: 表示主轴(Row为水平)的布局方向(阅读方向)
- .ltr: 中文、英语
- .rtl: 阿拉伯语、Hebrew
- verticalDirection: 表示纵轴(Row为垂直)的布局方向
- .up: 表示从底部到顶部, start在底部
- .down: 表示从顶部到底部, start在顶部
- textBaseline: 基线对齐
- children: 子组件数组
demo
Row和Column都只会在主轴方向占用尽可能大的空间,而纵轴的长度则取决于子组件长度中最大的, 比如在Row的高度, Column的宽度等
1 | Column( |
效果
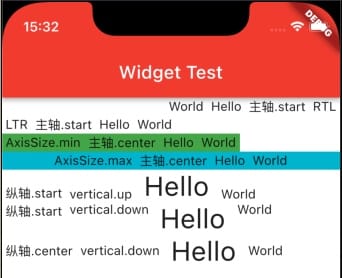
如果Row里面嵌套Row,或者Column里面再嵌套Column,那么只有最外面的Row或Column会占用尽可能大的空间,里面Row或Column所占用的空间为实际大小
1 | Container( |
效果:
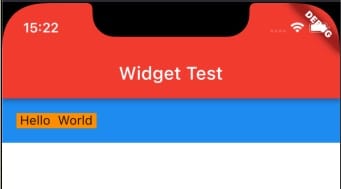